How to apply float precision (type specifier) in a conditional f-string?
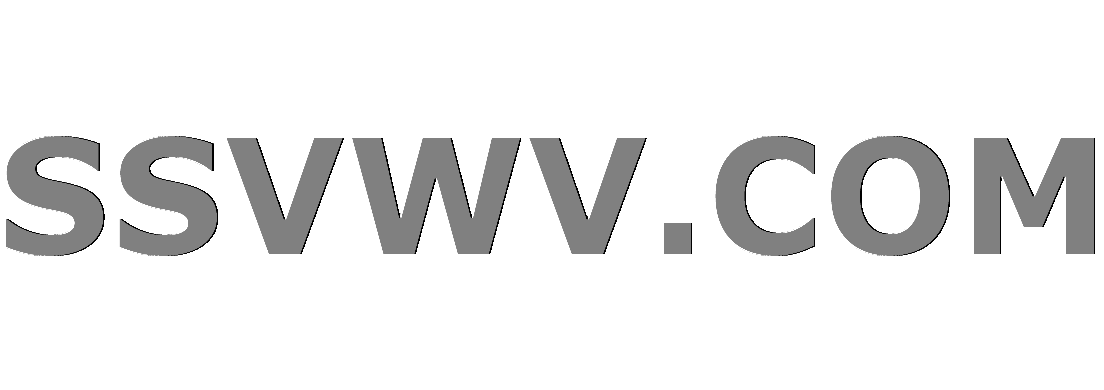
Multi tool use
I have the following f-string I want to print out on the condition the variable is available:
f"Percent growth: {self.percent_growth if True else 'No data yet'}"
Which results in:
Percent growth : 0.19824077757643577
So normally I'd use a type specifier for float precision like this:
f'{self.percent_growth:.2f}'
Which would result in:
0.198
But that messes with the if-statement in this case. Either it fails because:
f"Percent profit : {self.percent_profit:.2f if True else 'None yet'}"
The if statement becomes unreachable.
Or in the second way:
f"Percent profit : {self.percent_profit if True else 'None yet':.2f}"
The f-string fails whenever the condition leads to the else clause.
So my question is, how can I apply the float precision within the f-string when the f-string can result in two types?
python python-3.x f-string
add a comment |
I have the following f-string I want to print out on the condition the variable is available:
f"Percent growth: {self.percent_growth if True else 'No data yet'}"
Which results in:
Percent growth : 0.19824077757643577
So normally I'd use a type specifier for float precision like this:
f'{self.percent_growth:.2f}'
Which would result in:
0.198
But that messes with the if-statement in this case. Either it fails because:
f"Percent profit : {self.percent_profit:.2f if True else 'None yet'}"
The if statement becomes unreachable.
Or in the second way:
f"Percent profit : {self.percent_profit if True else 'None yet':.2f}"
The f-string fails whenever the condition leads to the else clause.
So my question is, how can I apply the float precision within the f-string when the f-string can result in two types?
python python-3.x f-string
1
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago
add a comment |
I have the following f-string I want to print out on the condition the variable is available:
f"Percent growth: {self.percent_growth if True else 'No data yet'}"
Which results in:
Percent growth : 0.19824077757643577
So normally I'd use a type specifier for float precision like this:
f'{self.percent_growth:.2f}'
Which would result in:
0.198
But that messes with the if-statement in this case. Either it fails because:
f"Percent profit : {self.percent_profit:.2f if True else 'None yet'}"
The if statement becomes unreachable.
Or in the second way:
f"Percent profit : {self.percent_profit if True else 'None yet':.2f}"
The f-string fails whenever the condition leads to the else clause.
So my question is, how can I apply the float precision within the f-string when the f-string can result in two types?
python python-3.x f-string
I have the following f-string I want to print out on the condition the variable is available:
f"Percent growth: {self.percent_growth if True else 'No data yet'}"
Which results in:
Percent growth : 0.19824077757643577
So normally I'd use a type specifier for float precision like this:
f'{self.percent_growth:.2f}'
Which would result in:
0.198
But that messes with the if-statement in this case. Either it fails because:
f"Percent profit : {self.percent_profit:.2f if True else 'None yet'}"
The if statement becomes unreachable.
Or in the second way:
f"Percent profit : {self.percent_profit if True else 'None yet':.2f}"
The f-string fails whenever the condition leads to the else clause.
So my question is, how can I apply the float precision within the f-string when the f-string can result in two types?
python python-3.x f-string
python python-3.x f-string
asked 4 hours ago
NoSplitSherlockNoSplitSherlock
1027
1027
1
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago
add a comment |
1
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago
1
1
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago
add a comment |
3 Answers
3
active
oldest
votes
You could use another f-string for your first condition:
f"Percent profit : {f'{self.percent_profit:.2f}' if True else 'None yet'}"
Admittedly not ideal, but it does the job.
1
Another way is usingstr.format
but I don't think it's any different.f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.
– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
@PatrickArtner see the OP’s question and code sample. Theif True
is a stand in for any conditional.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
add a comment |
You can use a ternary for the formatter as well - no need to stack 2 f-strings like Nikolas answer does:
for pg in (2.562345678, 0.9, None): # 0.0 is also Falsy - careful ;o)
print(f"Percent Growth: {pg if pg else 'No data yet':{'.05f' if pg else ''}}")
# you need to put '.05f' into a string for this to work and not complain
Output:
Percent growth: 2.56235
Percent growth: 0.90000
Percent growth: No data yet
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
add a comment |
I think the f string within f string answer is as simple as it gets, but if you want a little bit more readability, consider moving the condition outside the f string:
value = f'{self.percent_profit:.2f}' if True else 'No data yet'
print(f"Percent profit : {value}")
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54949918%2fhow-to-apply-float-precision-type-specifier-in-a-conditional-f-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could use another f-string for your first condition:
f"Percent profit : {f'{self.percent_profit:.2f}' if True else 'None yet'}"
Admittedly not ideal, but it does the job.
1
Another way is usingstr.format
but I don't think it's any different.f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.
– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
@PatrickArtner see the OP’s question and code sample. Theif True
is a stand in for any conditional.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
add a comment |
You could use another f-string for your first condition:
f"Percent profit : {f'{self.percent_profit:.2f}' if True else 'None yet'}"
Admittedly not ideal, but it does the job.
1
Another way is usingstr.format
but I don't think it's any different.f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.
– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
@PatrickArtner see the OP’s question and code sample. Theif True
is a stand in for any conditional.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
add a comment |
You could use another f-string for your first condition:
f"Percent profit : {f'{self.percent_profit:.2f}' if True else 'None yet'}"
Admittedly not ideal, but it does the job.
You could use another f-string for your first condition:
f"Percent profit : {f'{self.percent_profit:.2f}' if True else 'None yet'}"
Admittedly not ideal, but it does the job.
answered 4 hours ago
Nikolas Stevenson-MolnarNikolas Stevenson-Molnar
1,216714
1,216714
1
Another way is usingstr.format
but I don't think it's any different.f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.
– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
@PatrickArtner see the OP’s question and code sample. Theif True
is a stand in for any conditional.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
add a comment |
1
Another way is usingstr.format
but I don't think it's any different.f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.
– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
@PatrickArtner see the OP’s question and code sample. Theif True
is a stand in for any conditional.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
1
1
Another way is using
str.format
but I don't think it's any different. f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.– Idlehands
4 hours ago
Another way is using
str.format
but I don't think it's any different. f"Percent profit : {'{:.2f}'.format(self.percent_format) if True else 'None yet'}"
This is as simple as it gets if OP insists putting the condition within the f string itself.– Idlehands
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
@Idlehands Isn't it strange though that I can format strings and use conditionals, but not both at the same time?
– NoSplitSherlock
4 hours ago
1
1
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
@NoSplitSherlock If both outcomes of the the conditional were the same, you could. I think the tricky part of your example is that your conditional will yield either a float or a string.
– Nikolas Stevenson-Molnar
4 hours ago
1
1
@PatrickArtner see the OP’s question and code sample. The
if True
is a stand in for any conditional.– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner see the OP’s question and code sample. The
if True
is a stand in for any conditional.– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
@PatrickArtner Absolutely, that works too.
– Nikolas Stevenson-Molnar
3 hours ago
add a comment |
You can use a ternary for the formatter as well - no need to stack 2 f-strings like Nikolas answer does:
for pg in (2.562345678, 0.9, None): # 0.0 is also Falsy - careful ;o)
print(f"Percent Growth: {pg if pg else 'No data yet':{'.05f' if pg else ''}}")
# you need to put '.05f' into a string for this to work and not complain
Output:
Percent growth: 2.56235
Percent growth: 0.90000
Percent growth: No data yet
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
add a comment |
You can use a ternary for the formatter as well - no need to stack 2 f-strings like Nikolas answer does:
for pg in (2.562345678, 0.9, None): # 0.0 is also Falsy - careful ;o)
print(f"Percent Growth: {pg if pg else 'No data yet':{'.05f' if pg else ''}}")
# you need to put '.05f' into a string for this to work and not complain
Output:
Percent growth: 2.56235
Percent growth: 0.90000
Percent growth: No data yet
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
add a comment |
You can use a ternary for the formatter as well - no need to stack 2 f-strings like Nikolas answer does:
for pg in (2.562345678, 0.9, None): # 0.0 is also Falsy - careful ;o)
print(f"Percent Growth: {pg if pg else 'No data yet':{'.05f' if pg else ''}}")
# you need to put '.05f' into a string for this to work and not complain
Output:
Percent growth: 2.56235
Percent growth: 0.90000
Percent growth: No data yet
You can use a ternary for the formatter as well - no need to stack 2 f-strings like Nikolas answer does:
for pg in (2.562345678, 0.9, None): # 0.0 is also Falsy - careful ;o)
print(f"Percent Growth: {pg if pg else 'No data yet':{'.05f' if pg else ''}}")
# you need to put '.05f' into a string for this to work and not complain
Output:
Percent growth: 2.56235
Percent growth: 0.90000
Percent growth: No data yet
edited 3 hours ago
answered 3 hours ago


Patrick ArtnerPatrick Artner
24.9k62443
24.9k62443
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
add a comment |
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
1
1
I love how hacky this is.
– NoSplitSherlock
3 hours ago
I love how hacky this is.
– NoSplitSherlock
3 hours ago
add a comment |
I think the f string within f string answer is as simple as it gets, but if you want a little bit more readability, consider moving the condition outside the f string:
value = f'{self.percent_profit:.2f}' if True else 'No data yet'
print(f"Percent profit : {value}")
add a comment |
I think the f string within f string answer is as simple as it gets, but if you want a little bit more readability, consider moving the condition outside the f string:
value = f'{self.percent_profit:.2f}' if True else 'No data yet'
print(f"Percent profit : {value}")
add a comment |
I think the f string within f string answer is as simple as it gets, but if you want a little bit more readability, consider moving the condition outside the f string:
value = f'{self.percent_profit:.2f}' if True else 'No data yet'
print(f"Percent profit : {value}")
I think the f string within f string answer is as simple as it gets, but if you want a little bit more readability, consider moving the condition outside the f string:
value = f'{self.percent_profit:.2f}' if True else 'No data yet'
print(f"Percent profit : {value}")
answered 4 hours ago


IdlehandsIdlehands
5,6551620
5,6551620
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54949918%2fhow-to-apply-float-precision-type-specifier-in-a-conditional-f-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
D RPEvrmhfq qtIW TVrmkjlwQ2fxTQ BGc33,V4N,FzW,Be0pWoqP7X0dwFbrYe9B7Q,8oObJRejqAaQwoc,QzmD
1
I formulated the question this way so it was clear no matter what condition it fails to even execute.
– NoSplitSherlock
2 hours ago